Rails test jbuilder json response with rspec
Testing jbuilder response in rspec integration tests requires an extra helper method to be included in test. Requests in integration tests do not render json response unless we include render_views helper.
Today, while working on
rspec controller tests
for
jbuilder responses in Rails application,
the response for get
or post
requests was being returned as an empty string.
The setup for the rspec test was as given below. Let’s say we are showing post in json format using jbuilder.
Controller
class PostsController < ApplicationController
def show
@post = Post.find(params[:id])
end
end
JBuilder rendering json
View rendering json for the @posts
initialized in controller.
json.id @post.id
json.title @post.id
json.description @post.id
json.created_at @post.created_at
json.updated_at @post.updated_at
This will render json response as given below.
{
"id": 1,
"title": "Rails test jbuilder json response with rspec",
"description": "Testing jbuilder response in rspec integration tests requires an extra helper method to be included in test. Requests in integration tests do not render json response unless we include render_views helper.",
"created_at": "Thu, 11 Apr 2019 08:19:18 UTC +05:30",
"updated_at": "Thu, 11 Apr 2019 08:19:18 UTC +05:30"
}
Rspec Test
# frozen_string_literal: true
require 'rails_helper'
RSpec.describe PostsController, type: :controller do
context 'show' do
it 'should have required keys in the response json' do
# Let's say we have fixtures, to have post given below
post = posts(:jbuilder_test)
get :show, params: { id: post.id }, format: :json
end
end
end
As we can see, this test tries to get json response for the show action on posts controller.
If we inspect the repsonse, it is as given below.
$ response.body
# ""
This means jbuilder json response was not returned by the show
action
on
the posts_controller
.
render_views in tests
To be able to render json response from jbuilder view file, we need to add render_views.
Working rspec test with Jbuilder json
Now, the example can be written as given below.
# frozen_string_literal: true
require 'rails_helper'
RSpec.describe PostsController, type: :controller do
render_views
context 'show' do
it 'should have required keys in the response json' do
# Let's say we have fixtures, to have post given below
post = posts(:jbuilder_test)
get :show, params: { id: post.id }, format: :json
end
end
end
And running above rspec test will give the expected json response.
References
Subscribe to Ruby in Rails
Get the latest posts delivered right to your inbox
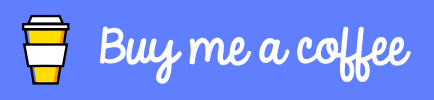