Split Rails routes into multiple files
Rails routes file can become lengthy if the application becomes huge. It can be difficult to manage routes especially if we have multiple namespace and route action names are similar across namespaces. Splitting Rails routes helps us manage routes easily in an application.
Rails routes file is available at config/routes.rb
by default.
It generally follows the pattern given below.
Rails.application.routes.draw do
# route 1
# route 2
# route .
# route .
# route N
end
As we can see, Rails.application.routes
calls method draw
by passing a block of routes defined in this file.
Rails.application.routes
is an instance of
ActionDispatch::Routing::RouteSet.
Let’s take an example config/routes.rb
file with some routes.
# config/routes.rb
Rails.application.routes.draw do
namespace :admin do
# ..
end
namespace :customer do
# ..
end
namespace :third_party do
# ..
end
end
To split this routes.rb
file into multiple files:
1. Create a directory config/routes
.
mkdir -p config/routes
2. Create new files in the config/routes
directory. In this case, admin_routes.rb
, customer_routes.rb
, third_party_routes.rb
# config/routes/admin_routes.rb
module AdminRoutes
def self.extended(router)
router.instance_exec do
# put admin namespace routes here
namespace :admin do
# ..
end
end
end
end
Similarly, define customer_routes.rb
and third_party_routes.rb
files.
# config/routes/customer_routes.rb
module CustomerRoutes
def self.extended(router)
router.instance_exec do
# put customer namespace routes here
namespace :customer do
# ..
end
end
end
end
# config/routes/third_party_routes.rb
module ThirdPartyRoutes
def self.extended(router)
router.instance_exec do
# put third_party namespace routes here
namespace :third_party do
# ..
end
end
end
end
Make sure these newly created route files are
auto loaded.
Add config/routes
directory files in autoload paths in config/application.rb
config.autoload_paths += Dir[ Rails.root.join(Rails.root.join('config', 'routes', '**/') ]
This auto loads modules defined in config/routes
directory and can be used in
config/routes.rb
file. Let’s load these routes in config/routes.rb
file as given below.
Rails.application.routes.draw do
extend AdminRoutes
extend CustomerRoutes
extend ThirdPartyRoutes
end
Run the rails application to verify that routes splitted in multiple files are loaded correctly and they work as expected.
Subscribe to Ruby in Rails
Get the latest posts delivered right to your inbox
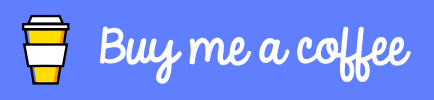