Rails 6 Parallel Tests
Rails 6 applications will run tests in parallel by default. The number of parallel workers to run tests can be customised. Moreover, all the parallel worker processes will have their own temporary database to run tests.
The feature is available for Rails 6+ applications. Rails applications having huge number of tests face the problem of huge runtime. Gems like Parallel Tests are used in older Rails applications to improve speed of test suite.
Need of Parallel testing in Rails
- Parallel testing is necessary in order to reducer the run time of test suite in Rails applications
- Tests run in single process don’t use multiple cores of the server (which are usually available these days!)
Setup
To be able to use parallel tests, call parallelize
helper in test_helper.rb
.
class ActiveSupport::TestCase
parallelize(workers: 3)
end
- This will split tests in 3 worker processes.
- Each worker will use it’s own temporary database to run tests
This can be configured in Rails 6+ applications with helper described above.
Setup and Teardown Hooks
Two hooks parallelize_setup
and parallelize_teardown
are provided.
parallelize_setup
hook is run after processes are forked, before tests are run.
This can be used to perform actions that need to be done before tests are run such as create databases.
parallelize_setup do
# create databases
end
parallelize_teardown
hook is run after tests are run.
This can be used to clean up e.g. drop database.
parallelize_teardown do
# drop databases
end
Parallel testing with processes / threads
class ActiveSupport::TestCase
parallelize(workers: 3)
end
When we set workers as described above, Rails 6 by default uses processes to run tests in parallel.
class ActiveSupport::TestCase
parallelize(workers: 3, with: :threads)
end
Pass an option with
and values as threads
to run tests in parallel using threads.
Find out more about process / thread option in Rails Guides
Customize number of parallel workers
We can customize number of worker processes to run by settings
an environment variable PARALLEL_WORKERS
.
PARALLEL_WORKERS=10 bin/rails test
- This will fork 10 processes and run tests in parallel.
- This will override default number set in
test_helper.rb
References
- Pull Request: Parallel Testing in Rails
- Rails Guides: Parallel Testing
Subscribe to Ruby in Rails
Get the latest posts delivered right to your inbox
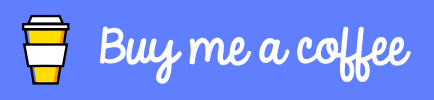