Rails Migration Change vs Up Down Methods
Rails Migrations have different ways of writing with change or up down methods. This tutorial will discuss exact difference between the both and what you should use for your migration. Rails Migration Change vs Up Down methods is important to be understood otherwise your migrations may behave in a way you might not want.
Example
Suppose we have users table in the database and we want to add new column to the table say,
country - the country of the user
This can be achieved in two ways in Migration -
1. Using change method
In Rails Migration there is new way of writing it as given below,
class AddCountryToUser < ActiveRecord::Migration
def change
add_column :users , :country, :string, :null => false # varchar field
end
end
####
What do we achieve by change?
1. We do not need to write specific down method if we use change method to write migrations
2. Rails automatically can detect what needs to be reverted considering what was changed in change method
3. Revert to this type of migrations works only when they are written by Rails Conventions only - like given above using, add_column, remove_column, create_table and so on.
4. When we try to revert, Rails will not be able to detect manual changes done in change method. e.g. If you alter database with
execute << SQL
way. So it will not get reverted if you perform revert of the migration
2. Using up down methods
In Rails Migration there is new way of writing it as given below,
class AddAbcColumnToUser < ActiveRecord::Migration
def self.up
add_column :users , :country, :string, :null => false # varchar field
end
def self.down
remove_column :users , :country, :string, :null => false # varchar field
end
end
####
What do we achieve by up down?
1. We need to define exclusively what should happen when running migration or reverting migration.
2. Only those changes defined by us in up/down methods will be performed by Rails.
3. We get more flexibility of defining behavior for manual updates of database -like we discussed,
execute << SQL
We can define specific revert action for such behavior
Conclusion
- We discussed what happens when we use change method and up and down method to write migrations.
- We should write migrations with change method if we are not migrating database with manual update, if we are - then we should consider using up down methods and write behavior that handles the manual update we want.
Reference
You can refer Migration for learning more about Rails Migrations.
Subscribe to Ruby in Rails
Get the latest posts delivered right to your inbox
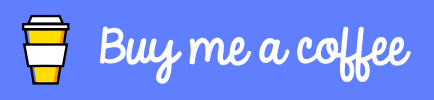